![]() |
![]() |
![]() |
![]() |
Integration Approaches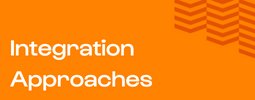
The Sugar platform is extremely powerful, flexible, and allows for easy integration. This flexibility means that determining the best approach for your use case scenario is the key to success.
- Push/Pull from Sugar via REST API
- Recommended approach
- Developers have full control over integration
- Integration can be stopped and systems will continue to work
- Retry mechanism: Resume integrations at any time (identify deltas through "last_update_time" flags)
- The time delay from/to Sugar is minimal
- Sugar REST API offers almost everything you need, and can be further extended via customization
- Push to Sugar, Sugar pushes to an external queue in realtime
- Use Sugar Events to trigger through Logic Hooks
- Requires Logic Hooks (code customization) to be created and deployed
- Does not offer a "retry mechanism", if a logic hook fails to deliver to a queue or contact an external system
- Synchronous in nature, requires a response from an external system
- Needs to handle dependencies manually (contact can only be created after account)
- Good to have a middleware/queue in front of Sugar to receive those event triggers to reliably manage peak loads
- Realtime: Embed your app into Sugar through Dashlets
- Simplest approach
- Often requires web user-based authentication
- All load/traffic goes directly to your system, which makes your system the performance bottleneck
REST API Webservices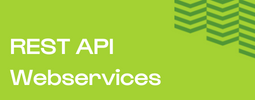
Accessing your data when you want is good. Accessing your data however you want is better. When you decide to build an integration with Sugar, you’ll need an easy way to access and interact with the data stored in Sugar. The REST (representational state transfer) API (application programming interface) is perfect for this.
- Sugar REST API Overview
- Fully RESTfull (GET, POST, PUT, DELETE)
- OAuth2 token based
- All fields and modules are available through the API automatically
- Metadata API provides everything you need to know about your Sugar instance.
- Topics
Common APIs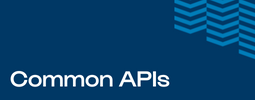
It is important to understand our most commonly used APIs and their functions within Sugar. Your integration might need data within Sugar's module structure that could potentially change over time, so you can use Metadata for it as well as CRUD onto Sugar.
- Metadata
- Endpoint with current system's structure
- Modules, fields, relationships, layouts
- Use carefully, its payload can be MBs in size, cache as much as possible
- GET /metadata (use filters as much as possible)
- It does not retrieve labels for dropdowns, use language API to do so
- 3 Tips for using the Sugar Metadata API
- Language
- Endpoint to retrieve labels
- Dropdown list values
- Any language-specific content
- GET /lang/<language_code>
- CRUD (Create, Read, Update, Delete) records
- Read:
GET /<module>/:record
- Create:
POST /<module>
- Update:
PUT /<module>/:record
- Delete:
DELETE /<module>/:record
- Filter:
POST /<module>/filter
- You can use GET as well
- POST solves the issue when a query is too long and hits HTTP limits
- Retrieve records related to another module:
GET /<module>/:record/link/:link_name
- Creates a relationship to a pre-existing record:
POST /<module>/:record/link/:link_name/:remote_id
- Read:
Performance and Best Practices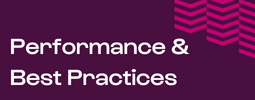
As you deep dive into Sugar APIs, you will see how easy it is to integrate to pull and push data from and to Sugar and your systems, however, you must consider performance so your integration doesn't become a bottleneck between those systems.
You should consider the following guidelines when implementing your integrations:
- Use Sugar's sync_key to link to remote IDs
- Sugar provides in every module a sync_key field
- sync_key is a unique identifier for that module that links back to your external system's generated key
- For example, account ID from ERP is AC0001, set sync_key (pass as a parameter in the POST) and sugar will create a record in account with sync_key=AC0001
- Next patch/update, sugar will check "where sync_key='AC0001'" and update it (it is indexed)
- Use Sugar Integrate Upsert APIs
- Sugar's "update or insert" API based on sync_key
- Simplifies a common operation - if record exists, update it, otherwise create it (GET followed by a PUT or POST)
- Upsert by sync_key
- Get by sync_key
- Delete by sync_key
- Set a sync on existing record (without sync_key)
- Create relationship based on sync_key
- Delete relationship based on sync_key
- Use Sugar Bulk API
- An endpoint that encapsulates multiple API calls into one single HTTP request
- Requests are executed synchronously (one after the other)
- Reducing network latency between calls
- Combined with Upsert provides the best performance
- Careful not to add too many requests to it
- Start small (5 to 10 requests) and increase until you reach its max capacity
- Polling and filtering time-based with Filter API
- Integration gateway (or your external system) asks Sugar every X minutes
- Request Filter API using
max_num
(max number of records), offset and fields - The response will provide the data as well as
next_offset
(offset can be seen as pagination) - if
next_offset
is -1, no more records to go through
- Initial Data Load into Sugar
- Use Bulk + Upsert to achieve data loads
- Millions of records may take a little while to process, plan ahead (over the weekend)
- Use multi-thread processes to load (for example 20 threads sending 20 bulk upsert requests)
- Careful with Sugar Cloud rate limits
- It's always a good idea to let Sugar Support know you're data loading, they will be considerate.
- Understanding PHP limitations
- PHP is single-threaded with limited resources to process your request
- If you send too much data using bulk, you may run out of memory for that request
- Push to the limits but give some room for PHP to finish the request gracefully
You're up and running, but don't stop now - this is just the beginning of your journey.
Now it's time for Customizations.